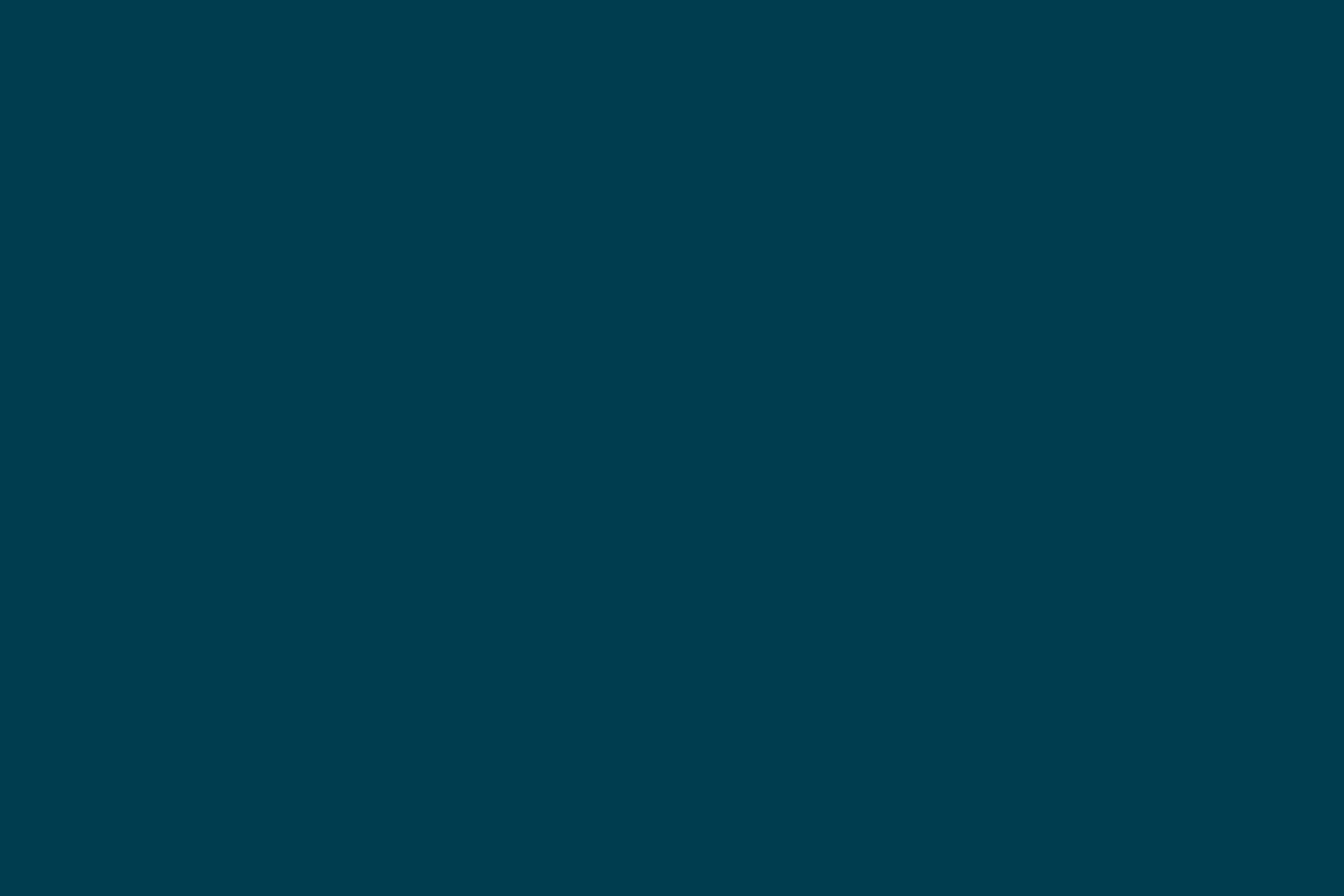
Introducing EMBD: A Superheroic Embedded Programming Framework
For the impatient, TheBot was an experiment to kickstart a hardware engineering culture at Thoughtworks. We choose the Raspberry Pi (RPi) as the prototyping platform and Golang as the language in which to create the firmware. We felt the need for a solid hardware abstraction layer (HAL) which would allow us to not only target the RPi, but soon expand to other platforms as well. We also wanted to make it dead easy to talk to a variety of sensors. Since there were no such existing frameworks for Golang, we ended up writing our own, and we called it EMBD (Github).
EMBD enables you to get started on your project quickly by providing built in support for the various platforms, protocols, sensors, controllers. It also allows your prototyping code to survive through to production because of the built in hardware abstraction layer and Golang’s versatility. Thus significantly shortening the time to market.
A Short History
A few months back, our Chairman, Roy Singham, asked Thoughtworkers to preempt the next big thing, so we naturally started looking at the Internet of Things (IoT) as one of the avenues for innovation. Approaching it from a purely hardware perspective would have meant a lot of playing catch up, as we would need to seed a lot of talent in the following spaces before we could catch up:
- material science
- industrial design
- manufacturing, etc...
We wanted to get started quickly, leveraging on abilities we have honed over the last two decades. And one of the low hanging fruits to explore was the software side of Internet-connected devices, firmware for the rovers and quadcopters, and the user experiences for these new contraptions.
Enter, TheBot
We deliberately choose to build something simple – to push the odds in our favor. However, the simplicity proved to be a boon as it allowed us a lot of scope for innovation in the software side of things. We also wanted to take this opportunity to study...to glean experiences from. From many angles, this is just scratching the surface of what is to come, but you have got to start somewhere. Think of it as bulb # 1.
(We first showcased this to public on Jan 10, 2014 and dedicated the effort to Aaron, a good soul and a Thoughtworker, who passed away on Jan 11, 2013.)
It was a lot of work. A lot of things you took for granted suddenly needed to be taken care of in code and in hardware. The fact that the code now had to actually “run” on the hardware brought in additional challenges as well. Concurrency is the name of the game. Most of the events which you needed to handle and react to (ex: a obstacle suddenly apprearing infront of the car, while the car is turning on its own) would come concurrently, in no particular guarenteed order. The firmware had to be both efficient and easy to reason about.
Golang to the Rescue
Created by a team at Google in 2007, Golang aimed at making software development pleasurable again. Software that built quickly, ran well on multi-core hardware in networked environments.
(see the official FAQs for a more complete picture)
Golang has excellent support for concurrency in the core language. The RaspberryPi is single threaded and we needed the car to handle multiple real world interactions at the same time. Using threads would have forced us to use mutexes, etc, for synchronization. The ‘goroutines+channels’ architecture in Golang helped us focus on the “actual” interactions. (Goroutines are light weight threads which are executed via the Go runtime on real threads via a M:N mapping. Channels are a typed mechanism for passing messages between goroutines). The resulting code is much easier to read, reason with and understand.
Simply running the binary was always enough. This helped tremendously in shortening our development/build/deploy cycles and made the process even more gratifying.
Golang is a statically typed, garbage collected and compiled programming language. However, in use, it feels like a FAST (slightly) verbose scripting language which has support for systems programming and duck typing. Since the cross compiled binary was entirely self contained, no runtime was needed. Simply running the binary was enough, which helped tremendously in shortening our development, build and deploy cycles and made the process even more gratifying.
(A recent article by SpaceMonkey details their story of switching from Python to Golang for the firmward of their embedded storage device. It's a must read to get a better idea of what Golang has to offer in this space.)
EMBD
EMBD is a framework for Go which does GPIO (General Purpose I/O), talks the I²C protocol (+ many more) and provides the drivers necessary to interact with many hardware sensors (eg. gyroscope, magnetometer, barometer, etc.) Its non-intrusive API allows you to quickly prototype solutions (see below for example) without sacrifising production-worthiness. And the best part? The code will run on a multitude of supported hosts (like the Raspberry Pi, and the BeagleBone Black) without change!
Although the framework started its life as go-rpi, we soon realized the potential of making it even more useful. The driver code we had written to talk to a plethora of sensors were not really dependent on the Raspberry Pi. They expected a I²C bus and not much else. So we immediately started thinking of ways in which we could allow people to leverage all that code in other platforms which Golang ran on (which turned out to be quite a few.)
We spent a bunch of time fine tuning the “feel” of the API. We have aimed to provide both real world usability (we did not want a toy feel to the API) and the ability to be be used for quick/rapid hardware prototyping.
For example, it leans towards rapid prototyping when needed:
func main() { for { embd.LEDToggle("LED0") time.Sleep(250 * time.Millisecond) } }
Or, gives you the control as necessary:
func main() { panicIf(embd.InitLED()) defer embd.CloseLED() led, err := embd.NewLED("LED0") if err != nil { panic(err) } defer led.Off() // Cleanly exit if someone hits Ctrl-C quit := make(chan os.Signal, 1) signal.Notify(quit, os.Interrupt, os.Kill) for { select { case <-time.After(250 * time.Millisecond): panicIf(led.Toggle()) fmt.Println("Toggled") case <-quit: return } } }
It currently has good support for the Raspberry Pi and the BeagleBone Black, with support for other platforms coming in the near future. This should almost guarentee that the particular combination of prototyping board, sensors, etc. would most probably work OOTB with EMBD.
EMBD is a bold attempt at creating a cross platform embedded programming library. A lot of work is needed to flesh out the sensor library and to bring in support for new hosts. The ROADMAP lists some of the short/long terms goals we have ahead of us, but we won’t get too far down that list without the support of the community. So looking forward to those pull requests!
Also, while we have done our best, if you do come across a bug, please let us know so that we can tackle it in the best way possible. And if you need any help, we will be hanging around here.
Karan is a developer with Thoughtworks India. This blog post first appeared on his website; view the original.
Links
Homepage: http://embd.kidoman.io/
Github: https://github.com/kidoman/embd
Disclaimer: The statements and opinions expressed in this article are those of the author(s) and do not necessarily reflect the positions of Thoughtworks.