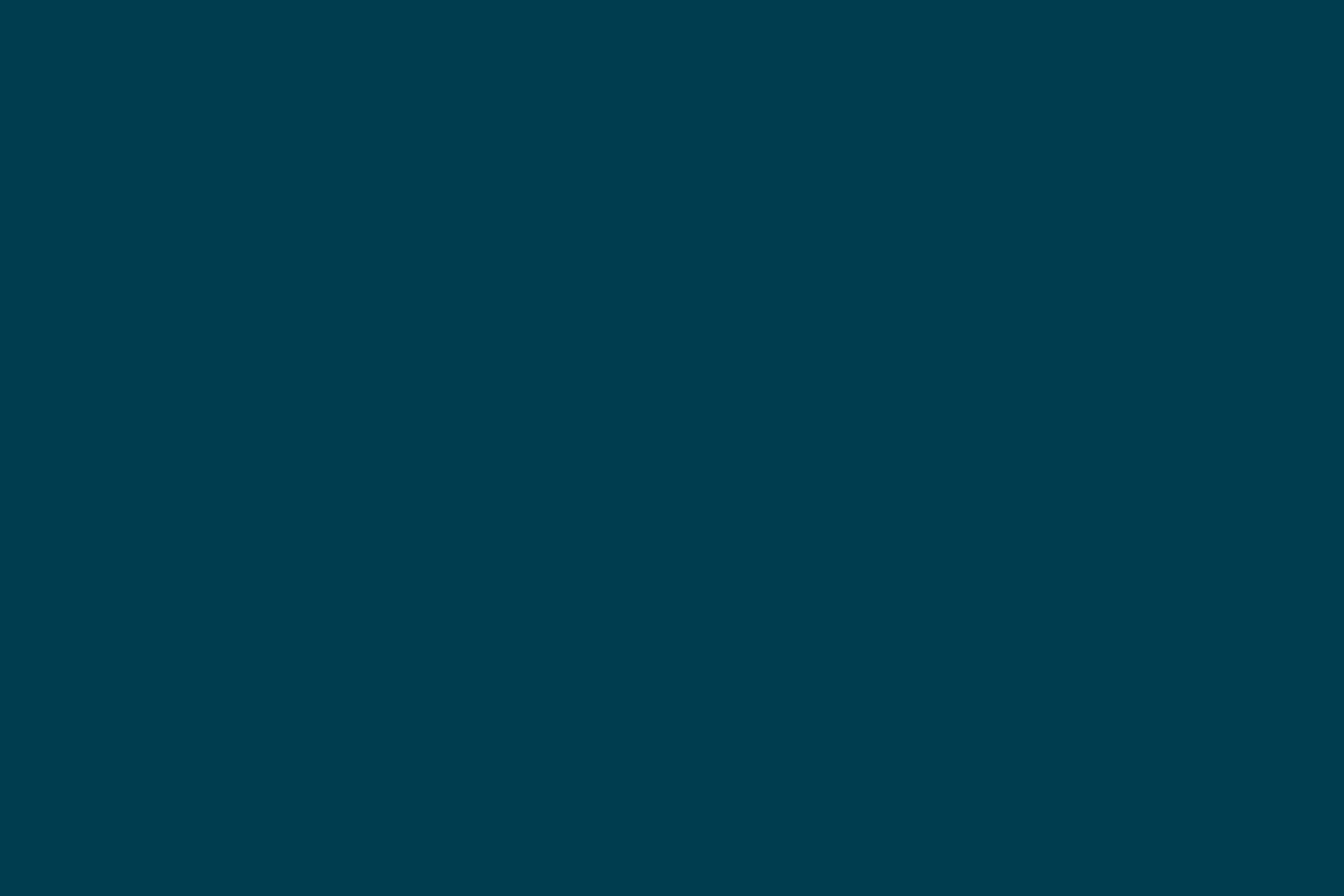
Testing native iOS applications with Twist
Twist supports native iOS application testing since version 13.3. Twist uses an open-source project called calabash
to enable native iOS testing. calabash
has support for both native and hybrid applications.
Getting started
Create a new Twist project with calabash driver.
While creating a project, you have to specify the XCode project location. Clicking Finish
will create a Twist project with calabash driver. Twist modifies the project's project.pbxproj
file and adds a calabash.framework
to the link path. This will be added to a new target with -cal
suffix. Eg: For an existing target named sample
, Twist will add a new target called sample-cal
. sample-cal
will have calabash.framework
linked to it. You need to build this target before running the tests.
calabash
works by altering your iOS application and embedding a HTTP server to it. This server will be started when the application is launched. The server component takes care of doing the actions specified by the Twist client.
Writing your first test
Twist has a fairly simple API for testing native iOS applications. An application is represented by the class IOSApplication
. Twist launches the application under test and provide an instance of IOSApplication
to the test implementation code. A typical Twist fixture would like the following.
Elements in the iOS application are represented by the class UIElement
. You can query using IOSApplication
to get a UIElement
. To query for a label with text foo
, you would write the code like:
UIElements uiElements = iosApplication.query("label marked:'foo'");
Above code returns a collection of UIElement
. Given a UIElement
, you can perform various actions on it. To perform a touch on the label:
UIElement label = iosApplication.query("label marked:'foo'").first();
label.touch();
Let us assume touching on label foo
, opens up a keyboard. Let us enter something to the current edit field.
UIElement label = iosApplication.query("label marked:'foo'").first();
label.touch();
iosApplication.awaitKeyboard();
iosApplication.getKeyboard().enterText("text entered using Twist");
iosApplication.getKeyboard().done();
Let us verify the text got entered correctly into the editable field.
assertTrue(iosApplication.elementExists("label marked:'text entered using Twist'"));
Now let us swipe the view and wait for the next screen to come up.
iosApplication.query("view").swipe(Direction.LEFT);
iosApplication.waitFor(new ICondition() {
@Override
public boolean test() throws CalabashException {
return iosApplication.elementExists("label marked:'in second screen'");
}
});
Same set of commands will work with UIElement
and UIElements
. When using UIElements
, Twist will act upon the first element available in the collection.
calabash
supports powerful query syntax. To learn more about all types of queries that you can perform, visit the calabash wiki.
Testing interactively
Most of the time, your application will have many elements and element hierarchies. It will be bit difficult to get an idea about how elements are laid out in the application. You need to know how elements are laid out and what are the accessibility properties it has to write efficient queries. Twist makes this process easier by introducing Application Spy
. Application spy helps you to get started with the API easily.
In the Twist IDE, select the iOS Twist project, click on the Spy icon to launch application spy. Application spy can also be launched by right clicking on the scenario editor and choosing Application spy
.
Spy looks like the following.
When you start application spy, it launches the application in a simulator/device (depending on the configuration), inspects the elements in that application and displays a hierarchical view of the entire application. Now it is very easy to understand how an application is laid out. With application spy, you can try out different commands interactively.
- You can enter a calabash query and get a hierarchical representation of the elements.
- You can click on the elements which are shown in the left pane of application spy and Twist will flash the respective element in the simulator/device.
- You can right click on an element and perform actions like,
touch
,swipe
etc on it. Twist will generate sample code for the action that you have performed. This code can be copied to the scenario implementation if required. - You can inspect element's default properties. New properties can be added if required.
Restarting application between scenarios
Twist won't restart the application after the scenario execution. If you need to restart the application after each scenario, you can write a context which does this.
Caveats
- No recorder available. You can use the code generated by application spy, but that is not a first class recording option.
- Application's source code should be available to perform testing. This is required for embedding the
calabash.framework
.
Disclaimer: The statements and opinions expressed in this article are those of the author(s) and do not necessarily reflect the positions of Thoughtworks.